Whenever you think of AI, normally you think about calling out to a server and doing the processing in the cloud, but there are seismic shifts happening with small models that can give your locally running applications a taste of those AI tools without the need for offloading everything to the cloud. One exciting example of this is the new Built-in AI APIs that Google has been experimenting with in Chrome and another is the open-source AiBrow extension which takes this a step further. Both are like having Ollama baked into your browser with native JavaScript APIs.
These new APIs allow developers to converse with the built-in browser AI, ask it to create summaries, write/rewrite text and translate text between languages. All of this AI goodness happens right within the browser, without anything leaving the machine or hitting servers in the cloud, giving developers a new tool to use in instances where privacy is paramount, network latency matters or where a built-in AI can give birth to new UX paradigms.
A new breed of web apps
Despite these APIs being in the early experimentation stage, Google is still encouraging developers to try them out and see what interesting use cases are out there, with their Built-in AI Challenge. After all, using the built-in browser AI isn't a case of moving all your code from the server onto the client, there are different constraints to think about, for example, it wouldn't make sense for everyone to summarize an email every time it's read.
Rethinking how you use AI running on the device brings new opportunities for web developers to create a new breed of web apps and bring functionality to the browser that simply wasn't possible before. This could be something as exotic as questioning a long PDF document for the information you need, automatically categorizing some text based on previously seen categories or extracting structured information from any piece of text.
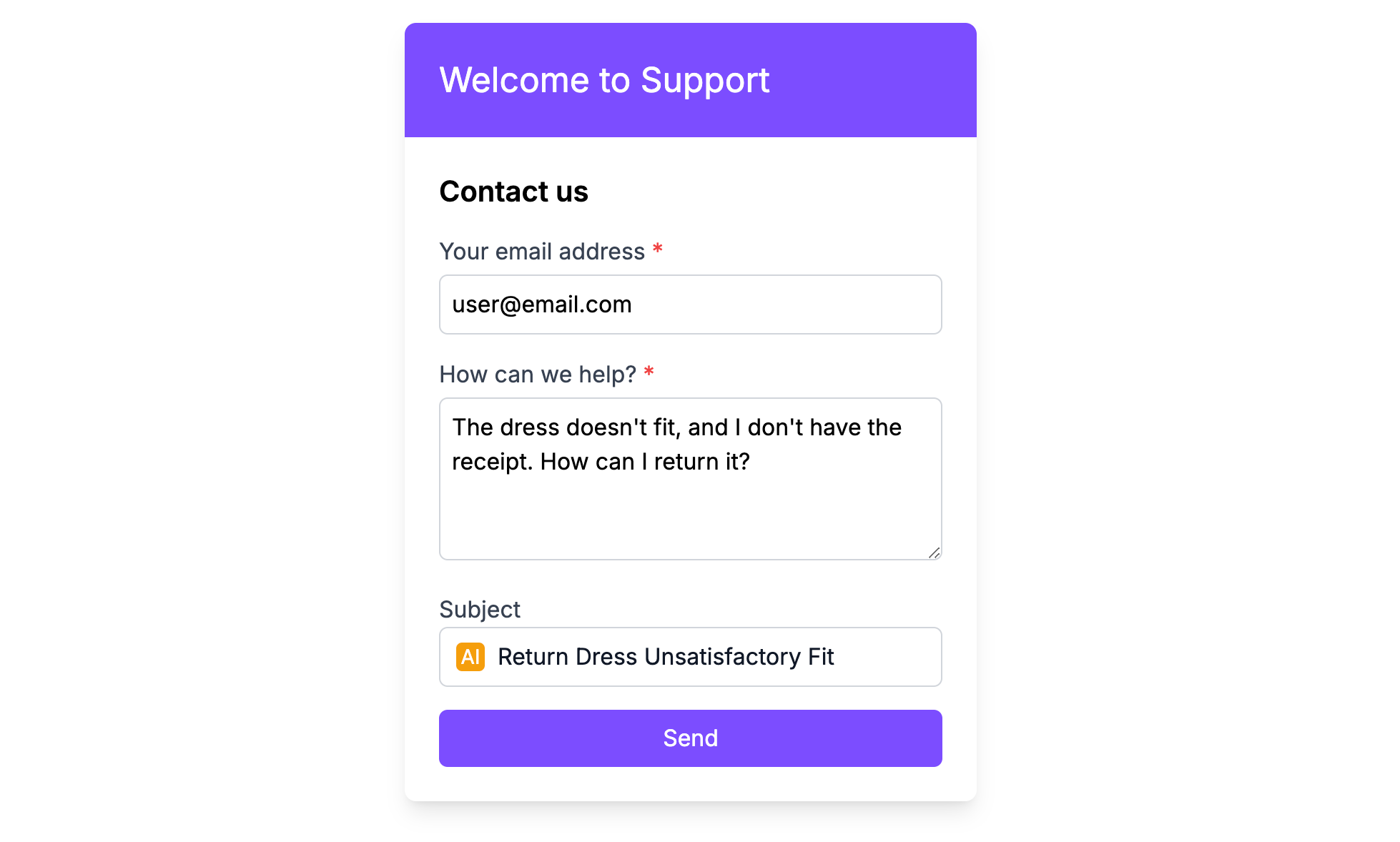
Expanding the scope of built-in AI
Google is still in the experimentation stage and we think this is an excellent time to look at the shape of those APIs, what they can do and what it might mean for developers. That's why we started experimenting with AiBrow, an open-source browser extension which expands on the capabilities of what the Chrome APIs are able to do with more tooling like Grammar support, Embeddings, different models and more.
We also wanted to look at what support for other browsers might look like, so AiBrow polyfills the current set of APIs for other Chromium browsers like Edge, Brave, Wavebox & Vivaldi as well as Firefox. If you're looking into the future of developing with AI, you know that you can count on broad browser support.
Building with built-in AI
To start developing with built-in AI, you should install the AiBrow extension and take a look at the developer docs. Once AiBrow is installed, you can run it from any page and use it to start generating text...
if (window.aibrow) {
const { helper } = await window.aibrow.capabilities()
if (helper) {
const session = await window.aibrow.languageModel.create()
const stream = await sess.promptStreaming('Write a 3 verse poem about AI in the browser')
for await (const chunk of stream) {
console.log(chunk)
}
} else {
console.log('Aibrow helper not installed')
}
} else {
console.log('Aibrow not installed')
}
Check that AiBrow is installed and then ask it to write a poem
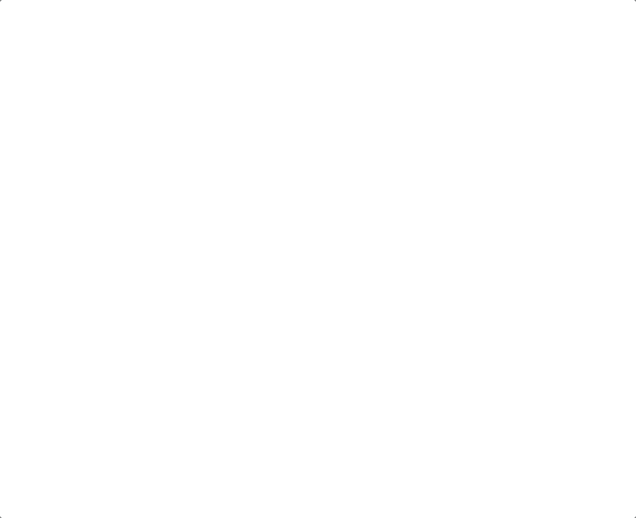
What can it do?
The AiBrow API is split into multiple top-level categories. Those that are available in Chrome are API compatible, meaning you can use the Chrome implementation or the AiBrow implementation interchangeably
window.aibrow.languageModel
📒 Documentation | Demo | ✅ Chrome implementation
The languageModel API allows you to chat with the built-in device model by sending messages and receiving replies in a conversational format.
window.aibrow.summarizer
📒 Documentation | Demo | ✅ Chrome implementation
Use the summarizer API to summarize meeting transcripts, give a sentence or paragraph-sized summary of product reviews, summarize long articles or generate article titles.
window.ai.rewriter
📒 Documentation | Playground | ✅ Chrome implementation
Use the rewriter API to change the length of some text, change the formality, rephrase text or change it to use simpler words and concepts (i.e. explain like I'm 5)
window.ai.writer
📒 Documentation | Playground | ✅ Chrome implementation
Use the writer API to generate textual explanations of structured data, expand pro/con lists, break through writer's block and create a first draft of blog articles.
window.ai.translator
📒 Documentation | ✅ Chrome implementation
Use the translation API to translate a block of text
window.ai.languageDetector
📒 Documentation | ✅ Chrome implementation
Use the language detector API to detect the language of some text.
window.ai.embedding
📒 Documentation | 🆕 AiBrow experiement
AiBrow allows you to create embeddings from any piece of text. These can then be stored and searched over to find similar text to a new input
window.ai.coreModel
📒 Documentation | 🆕 AiBrow experiement
The CoreModel API is powerful because it exposes the underlying model to the API and passes your prompts and options directly through. This gives you more power to constrain the language model in new ways, with the added complexity of building templates and configurations yourself.
Who can use it?
To use the built-in Chrome APIs, you either need to enable browser flags or register your site for an origin trial. More details. Alternatively, you can install the AiBrow extension for your browser and start coding with AI right away.
If you're using AiBrow on the web, you can start using the window.aibrow
APIs immediately from any page. If you want to use AiBrow in an extension, there's a hand npm library that allows you to import all the AiBrow APIs.
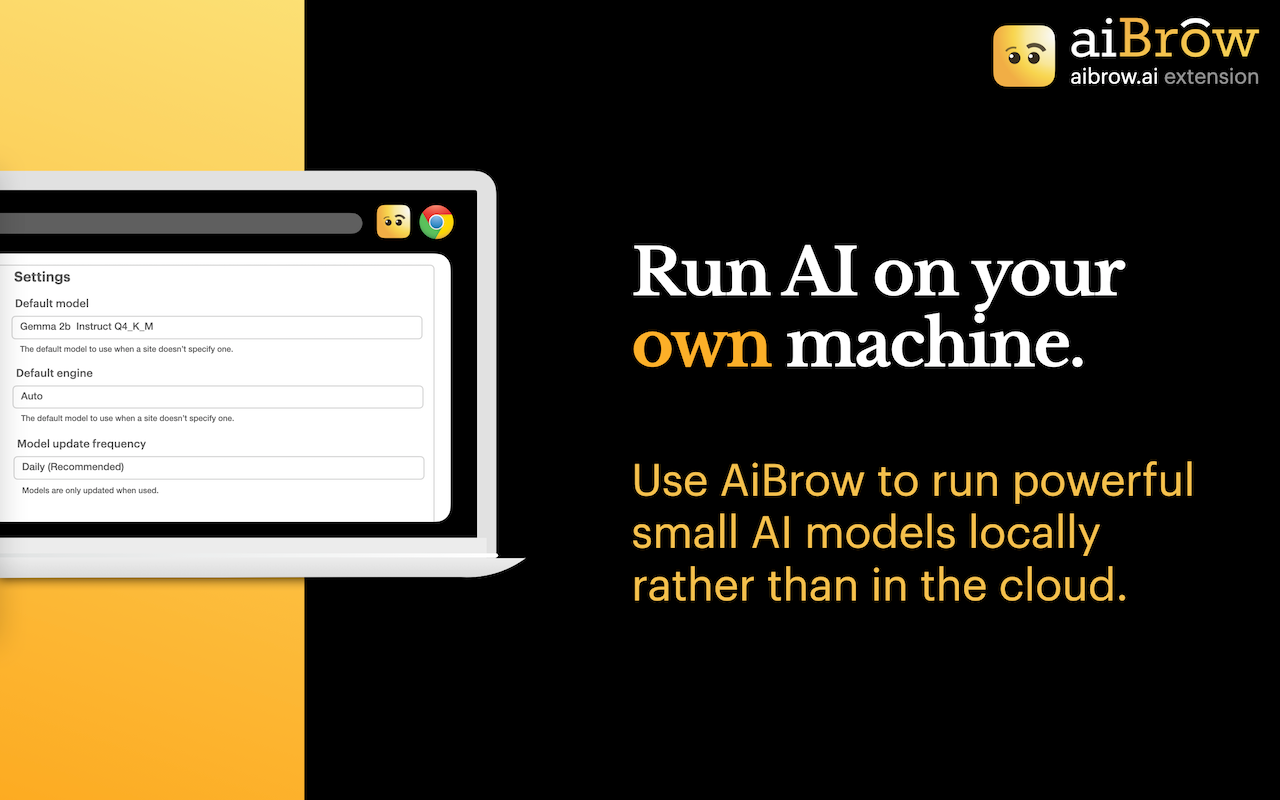